Introduction
Today, I am explaining about cascading drop down list in MVC, while we are working on CRM, ERP, Web Apps or other web apps then we often require to populate cascading dropdown list e.g. First populate Country then select country from list then corresponding state will be populated in other dropdown. This is the way that how we need cascading dropdown list in our MVC applications. Here I am taking example, first populating drop down list of classes, on the click of classes, the other drop down list will be populated of Subjects and then while we click on Subjects then chapter will be populated correspondingly.Add Entity Framework Model in MVC Project
First of all, you have to add entities in our project to populate cascading drop down in our project. I am providing a video link (Add Entities) which explains all steps how to add entities in our project using entity framework.namespace MvcApplication1.Context { using System; using System.Data.Entity; using System.Data.Entity.Infrastructure; public partial class MVC_AppEntities1 : DbContext { public MVC_AppEntities1() : base("name=MVC_AppEntities1") { } protected override void OnModelCreating(DbModelBuilder modelBuilder) { throw new UnintentionalCodeFirstException(); } public virtual DbSettblChapterMasters { get; set; } public virtual DbSet tblClassMasters { get; set; } public virtual DbSet tblSubjectMasters { get; set; } } }
Populate First Dropdown List (Subjects)
Here I have written an action method in controller as below:[HttpGet] public ActionResult My_MVC_App(string ddlClasses, string ddlSubjects, string ddlChapters) { var _context = new MVC_AppEntities1(); List_classlst = GetClasses(); // Clases List li = new List (); foreach (var item in _classlst) { li.Add(new SelectListItem { Text = item.ClassName, Value = item.ClassIDPK.ToString() }); } ViewData["Classes"] = li; return View(); }
Populate Second Dropdown List (Subjects)
Next step is to write action method to populate second dropdown list of subject while we click on classes drop down list.[HttpGet] public JsonResult GetSubjectsNew(Int16 ClassID) { List_lstSubjects = GetSubjectsID(ClassID); List _liSubj = new List (); /// var _context = new MVC_AppEntities1(); foreach (var _itemsubject in _lstSubjects) { _liSubj.Add(new SelectListItem { Text = _itemsubject.SubjectName, Value = _itemsubject.SubjectIDPK.ToString() }); } return Json(new SelectList(_liSubj, "Value", "Text"), JsonRequestBehavior.AllowGet);
Populate Third Dropdown List (Chapter)
Now next step is to populate third dropdown list of chapters while we click on subjects correspondingly.[HttpGet] public JsonResult GetChapterList(Int16 SubjectID, Int32? ChapterID) { List_lstChapters = GetChapters(SubjectID); List _liSubj = new List (); foreach (var _itemsubject in _lstChapters) { _liSubj.Add(new SelectListItem { Text = _itemsubject.ChapterName, Value = _itemsubject.ChapterIDPK.ToString() }); } return Json(new SelectList(_liSubj, "Value", "Text"), JsonRequestBehavior.AllowGet); }
Helping Method to Populate All Dropdowns (Classes, Subjects, Chapters)
MVC Razor View
Import Jquery Library in our Project (Under Head Tag)
MVC Razor HTML Helpers
Below is written code to provide complete interface to populate three dropdowns.Jquery Ajax call to MVC Controller
Here is written a Ajax/Jquery method to call Controller action for for subjectsHere is written other Ajax/Jquery method to call Controller action for the chapters as below:
Conclusion
Explained above about cascading dropdown in MVC with concerned every aspect of the MVC apps. Cascading dropdown is the common requirement of our each MVC applications, if we skip few steps to cascading drop down then sometimes it waste our very precious time of development.Development Environment
Visual Studio 2013, Entity Framework 6.0, Database script under app folder App_Data, Change web.config settings according to your machine specific, Data source, Initial Catalog, Database User ID, Password etc.Download Complete Project
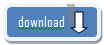
Ajax in MVC is very important and nice explanation for it..
ReplyDeleteASP.Net MVC Training
Online MVC Training
Online MVC Training from India
MVC Training in Chennai
Dot Net Framework
IT Technical Articles